我们在之前介绍了一篇关于PE文件结构的文章,其中描述了各个段的参数,那这篇文章就来尝试介绍通过代码来获取对应文件的参数。
因为考虑到方法的多样性,我们来约束几个条件,首先,我们尝试用go语言来获取,因为方便后面以可视化的方式呈现(见之前介绍的一篇文章https://daliu.net/posts/20250113/),其次,关于文件的形式,我们可以尝试获取静态文件,以及加载到内存中的文件的相关重点参数。
关于pe文件的参数,我们在之前的一篇文章中做了详细的介绍,https://daliu.net/posts/20250117/。我们把关于PE文件各结构的情况做了清晰的展示,那么我们接下来常用用代码解析一个静态文件,但是本次用go语言来编程,如果用go的话,其实这个问题反而就异常简单了,因为go有很多库,会很方便的进行解析pe文件。
我们本次使用的库就是“github.com/Binject/debug/pe”这个库,先说下我们如何找到然后再展示使用方法:
首先,在搜索引擎随便搜索下就能知道,go有一个标准库是debug/pe,我们可以去官方文档看下,访问https://pkg.go.dev/,在里面搜索pe,会发现有两个包,一个是debug/pe,还有一个是github.com/Binject/debug/pe 我们通过搜索可以看到如下图片:
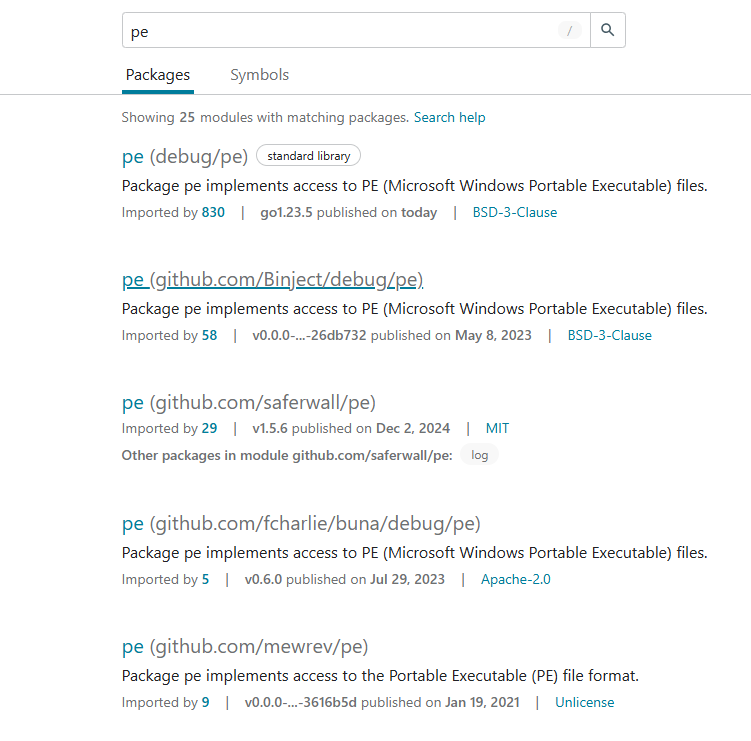
点进第一个标准库就可以看到这个库提供了哪些关于pe的结构体和方法,而标准库的方法比较少,我们可以看第二个库,这个库的方法就稍稍多一些,至于行不行,直接来测一测就可以了。
首先我们先把这个库引入:依然是在vscode中,新建终端,终端中输入go get github.com/Binject/debug/pe
,然后在go的文件中,import这个库
pe文件里的结构体就是file,所以,我们可以直接用这个结构体。
如果已经安装好并引入了这个库,那么获取导出表可以直接通过其中的方法获取,代码如下:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
|
package main
import (
"fmt"
"log"
"github.com/Binject/debug/pe"
)
func main() {
ntdll, error := pe.Open("c:\\windows\\system32\\ntdll.dll")
if error != nil {
log.Fatal(error)
}
// 获取导出表信息
exps, err := ntdll.Exports()
if err != nil {
log.Fatal(err)
}
// 遍历导出表并打印
for _, exp := range exps {
fmt.Printf("name:%s rva:%x ordinal:%d \n ", exp.Name, exp.VirtualAddress, exp.Ordinal)
}
}
|
最后打印的效果如下:
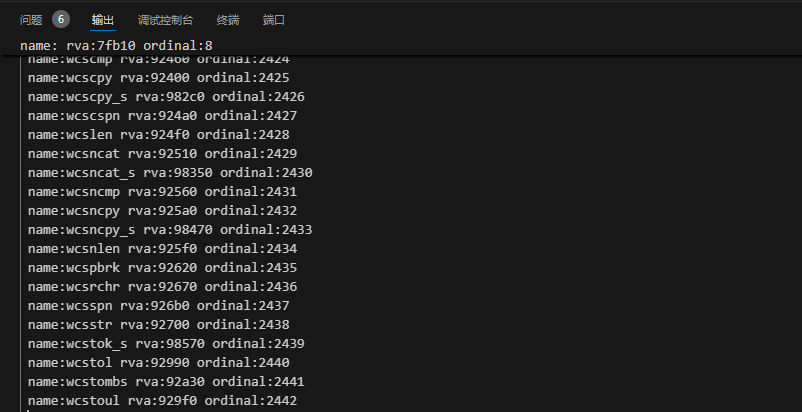
我们再用IDA打开同一文件比对一下,是一样的。
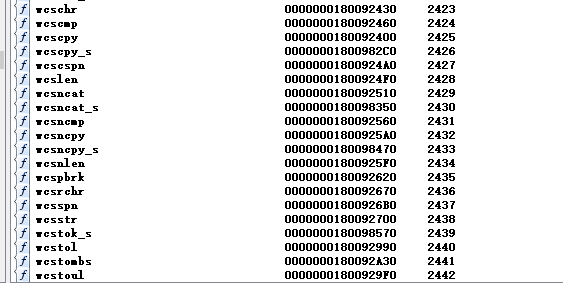
然后我们获取导入表,导入表的获取没有更特定的函数,有一个方法是获取导入描述表的,也就是_IMAGE_IMPORT_DESCRIPTOR 这个结构体的,获取之后,获取之后我们还需要通过OriginalFirstThunk来进一步获取每一个导入结构的函数名。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
typedef struct _IMAGE_IMPORT_DESCRIPTOR {
union {
DWORD Characteristics; // 0 for terminating null import descriptor
DWORD OriginalFirstThunk; // RVA to original unbound IAT (PIMAGE_THUNK_DATA)
} DUMMYUNIONNAME;
DWORD TimeDateStamp; // 0 if not bound,
// -1 if bound, and real date\time stamp
// in IMAGE_DIRECTORY_ENTRY_BOUND_IMPORT (new BIND)
// O.W. date/time stamp of DLL bound to (Old BIND)
DWORD ForwarderChain; // -1 if no forwarders
DWORD Name;
DWORD FirstThunk; // RVA to IAT (if bound this IAT has actual addresses)
} IMAGE_IMPORT_DESCRIPTOR;
|
因为是内存文件,没有导入函数的地址,所以我们就获取OriginalFirstThunk并尝试获取函数的名称,关键代码如下:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
|
// 获取文件字节数据
ntdllBytes, error := ntdll.Bytes()
//获取_IMAGE_IMPORT_DESCRIPTOR
importDes, sec, _, error := ntdll.ImportDirectoryTable()
// 先将OriginalFirstThunk这个RVA转换为文件偏移量
oftFoa := ntdll.RVAToFileOffset(imp.OriginalFirstThunk)
// 通过偏移获取truck指定地址的数据
importNameorOrdinal := binary.LittleEndian.Uint32(ntdllBytes[oftFoa : oftFoa+4])
// 判断是否最高位为1,是按照序号导入还是名称导入
if importNameorOrdinal&0x80000000 != 0 {
fmt.Printf("序号:%d \n ", binary.LittleEndian.Uint16(ntdllBytes[oftFoa:oftFoa+2]))
} else {
funcNameAddrFoa := ntdll.RVAToFileOffset(importNameorOrdinal)
// 获取函数名称的结束位置
funcNameEndAddrFoa := funcNameAddrFoa
funcNameEndAddrFoa = funcNameEndAddrFoa + 2
for ntdllBytes[funcNameEndAddrFoa] != 0 {
funcNameEndAddrFoa++
}
fmt.Printf("函数名:%s \n ", string(ntdllBytes[funcNameAddrFoa+2:funcNameEndAddrFoa]))
}
|
为了我们可以循环获取每一个_IMAGE_IMPORT_DESCRIPTOR,然后再每一个_IMAGE_IMPORT_DESCRIPTOR描述的truck继续遍历,获取每一个导入函数的名称或序号,为此还需要判断是32位还是64位,因为不同程序对应的truck的大小不同,所以要分开处理,整体的代码如下:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
|
package main
import (
"encoding/binary"
"fmt"
"log"
"github.com/Binject/debug/pe"
)
func main() {
// ntdll, error := pe.Open("D:\\kernel_study\\x86\\ntoskrnl.exe")
ntdll, error := pe.Open("D:\\source\\credetial-provider-test\\x64\\Release\\http-test.exe")
if error != nil {
log.Fatal(error)
}
// 获取文件字节数据
ntdllBytes, error := ntdll.Bytes()
if error != nil {
log.Fatal(error)
}
//获取_IMAGE_IMPORT_DESCRIPTOR
importDes, sec, _, error := ntdll.ImportDirectoryTable()
if error != nil {
log.Fatal(error)
}
fmt.Printf("sectionName:%s\n ", sec.Name)
// 获取计算机类型
marchineType := ntdll.FileHeader.Machine
// 通过获取到的导入表的OriginalFirstThunk获取导入函数的名称或序号
for _, imp := range importDes {
fmt.Printf("name:%s rva:%x \n ", imp.DllName, imp.OriginalFirstThunk)
// 先将OriginalFirstThunk这个RVA转换为文件偏移量
oftFoa := ntdll.RVAToFileOffset(imp.OriginalFirstThunk)
if marchineType == 0x8664 {
// 循环读取导入表中的数据,判断是序号还是名称
importNameorOrdinal := binary.LittleEndian.Uint64(ntdllBytes[oftFoa : oftFoa+8])
for importNameorOrdinal != 0 {
if importNameorOrdinal&0x8000000000000000 != 0 {
fmt.Printf("序号:%d \n ", binary.LittleEndian.Uint16(ntdllBytes[oftFoa:oftFoa+2]))
} else {
funcNameAddrFoa := ntdll.RVAToFileOffset(binary.LittleEndian.Uint32(ntdllBytes[oftFoa : oftFoa+4]))
// 获取函数名称的结束位置
funcNameEndAddrFoa := funcNameAddrFoa
funcNameEndAddrFoa = funcNameEndAddrFoa + 2
for ntdllBytes[funcNameEndAddrFoa] != 0 {
funcNameEndAddrFoa++
}
fmt.Printf("函数名:%s \n ", string(ntdllBytes[funcNameAddrFoa+2:funcNameEndAddrFoa]))
}
oftFoa += 8
importNameorOrdinal = binary.LittleEndian.Uint64(ntdllBytes[oftFoa : oftFoa+8])
}
} else {
// 通过偏移获取truck指定地址的数据
importNameorOrdinal := binary.LittleEndian.Uint32(ntdllBytes[oftFoa : oftFoa+4])
// 循环读取导入表中的数据,判断是序号还是名称
for importNameorOrdinal != 0 {
if importNameorOrdinal&0x80000000 != 0 {
fmt.Printf("序号:%d \n ", binary.LittleEndian.Uint16(ntdllBytes[oftFoa:oftFoa+2]))
} else {
funcNameAddrFoa := ntdll.RVAToFileOffset(importNameorOrdinal)
// 获取函数名称的结束位置
funcNameEndAddrFoa := funcNameAddrFoa
funcNameEndAddrFoa = funcNameEndAddrFoa + 2
for ntdllBytes[funcNameEndAddrFoa] != 0 {
funcNameEndAddrFoa++
}
fmt.Printf("函数名:%s \n ", string(ntdllBytes[funcNameAddrFoa+2:funcNameEndAddrFoa]))
}
oftFoa += 4
importNameorOrdinal = binary.LittleEndian.Uint32(ntdllBytes[oftFoa : oftFoa+4])
}
}
}
}
|
上文代码中,marchineType这个参数是在FileHeader中的参数,标识计算机,映像文件只能在指定计算机或模拟指定计算机的系统上运行,具体参考官方文档可得到对应介绍https://learn.microsoft.com/zh-cn/windows/win32/debug/pe-format,0x8664标识64位,0x14c为Intel 386 或更高版本的处理器和兼容的处理器。
运行起来,最后得到的效果如图所示,这样我们就得到了导入表的信息。
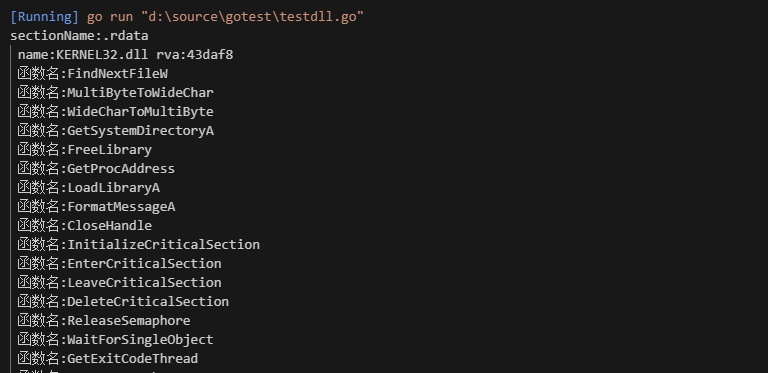
重定位表跟导出表一样,直接就保存在了结构体中,可以直接拿出来遍历,代码如下:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
|
package main
import (
"encoding/binary"
"fmt"
"log"
"github.com/Binject/debug/pe"
)
func main() {
//ntdll, error := pe.Open("D:\\kernel_study\\x86\\ntoskrnl.exe")
ntdll, error := pe.Open("D:\\source\\credetial-provider-test\\x64\\Release\\http-test.exe")
if error != nil {
log.Fatal(error)
}
//获取文件字节数据
ntdllBytes, error := ntdll.Bytes()
if error != nil {
log.Fatal(error)
}
// 遍历重定位表
for _, entry := range *ntdll.BaseRelocationTable {
fmt.Printf("VirtualAddress:%x, SizeOfBlock:%d\n ", entry.VirtualAddress, entry.SizeOfBlock)
for _, item := range entry.BlockItems {
if item.Type == 0 {
continue
}
if item.Type == 3 || item.Type == 10 {
relocItemAddrRva := entry.VirtualAddress + uint32(item.Offset)
relocItemAddrFoa := ntdll.RVAToFileOffset(relocItemAddrRva)
fmt.Printf("Type:%x, relocItemAddrRva:%x relocItemAddrFoa:= %x\n ", item.Type, relocItemAddrRva, relocItemAddrFoa)
// 读取偏移量处的数据
if item.Type == 3 {
relocItemAddr := binary.LittleEndian.Uint32(ntdllBytes[relocItemAddrFoa : relocItemAddrFoa+4])
fmt.Printf("relocItemAddr:%x\n ", relocItemAddr)
} else if item.Type == 10 {
relocItemAddr := binary.LittleEndian.Uint64(ntdllBytes[relocItemAddrFoa : relocItemAddrFoa+8])
fmt.Printf("relocItemAddr:%x\n ", relocItemAddr)
}
}
}
}
}
|
遍历的结果如图所示:
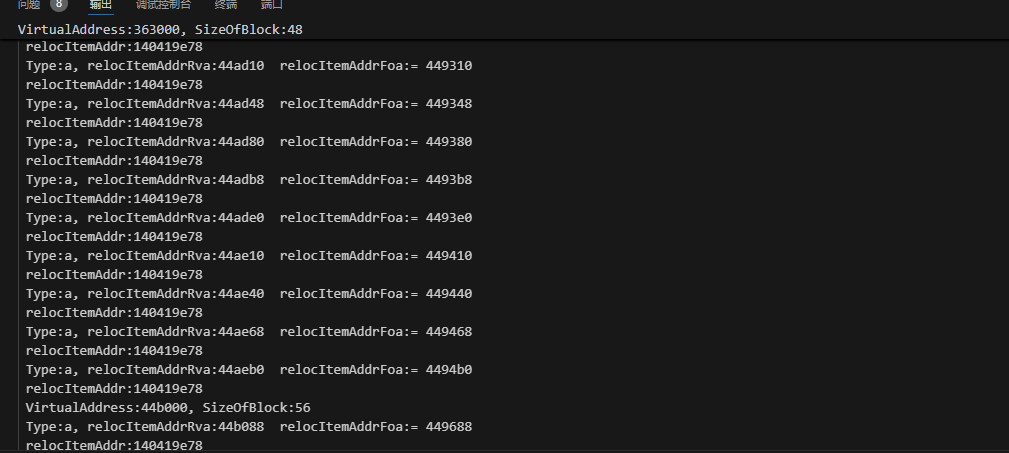
其实刚刚介绍的pe的库是可以获取动态文件的参数的,因为它可以通过内存中的地址来获取实例化file结构体,不过有一个前提,就是需要获取加载到内存中的pe文件的基址,假设我们需要获取当前进程的pe文件的基址,通过刚刚的库我们似乎就无能为力了,但是别忘记我们还有windows的API函数呀,我们可以利用windows提供的API来获取对应的目标模块的基址。
如果想获取当前进程的基址,就要知道进程的信息,而进程的信息一般存放在一个叫做PEB的结构里,也就是进程环境块,官方文档如下:https://learn.microsoft.com/zh-cn/windows/win32/api/winternl/ns-winternl-peb里面省略了好多,展开信息的话可以看下面的结构:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
|
//0x7c8 bytes (sizeof)
struct _PEB
{
UCHAR InheritedAddressSpace; //0x0
UCHAR ReadImageFileExecOptions; //0x1
UCHAR BeingDebugged; //0x2
union
{
UCHAR BitField; //0x3
struct
{
UCHAR ImageUsesLargePages:1; //0x3
UCHAR IsProtectedProcess:1; //0x3
UCHAR IsImageDynamicallyRelocated:1; //0x3
UCHAR SkipPatchingUser32Forwarders:1; //0x3
UCHAR IsPackagedProcess:1; //0x3
UCHAR IsAppContainer:1; //0x3
UCHAR IsProtectedProcessLight:1; //0x3
UCHAR IsLongPathAwareProcess:1; //0x3
};
};
UCHAR Padding0[4]; //0x4
VOID* Mutant; //0x8
VOID* ImageBaseAddress; //0x10
struct _PEB_LDR_DATA* Ldr; //0x18
struct _RTL_USER_PROCESS_PARAMETERS* ProcessParameters; //0x20
VOID* SubSystemData; //0x28
VOID* ProcessHeap; //0x30
struct _RTL_CRITICAL_SECTION* FastPebLock; //0x38
union _SLIST_HEADER* volatile AtlThunkSListPtr; //0x40
VOID* IFEOKey; //0x48
union
{
ULONG CrossProcessFlags; //0x50
struct
{
ULONG ProcessInJob:1; //0x50
ULONG ProcessInitializing:1; //0x50
ULONG ProcessUsingVEH:1; //0x50
ULONG ProcessUsingVCH:1; //0x50
ULONG ProcessUsingFTH:1; //0x50
ULONG ProcessPreviouslyThrottled:1; //0x50
ULONG ProcessCurrentlyThrottled:1; //0x50
ULONG ProcessImagesHotPatched:1; //0x50
ULONG ReservedBits0:24; //0x50
};
};
UCHAR Padding1[4]; //0x54
union
{
VOID* KernelCallbackTable; //0x58
VOID* UserSharedInfoPtr; //0x58
};
ULONG SystemReserved; //0x60
ULONG AtlThunkSListPtr32; //0x64
VOID* ApiSetMap; //0x68
ULONG TlsExpansionCounter; //0x70
UCHAR Padding2[4]; //0x74
VOID* TlsBitmap; //0x78
ULONG TlsBitmapBits[2]; //0x80
VOID* ReadOnlySharedMemoryBase; //0x88
VOID* SharedData; //0x90
VOID** ReadOnlyStaticServerData; //0x98
VOID* AnsiCodePageData; //0xa0
VOID* OemCodePageData; //0xa8
VOID* UnicodeCaseTableData; //0xb0
ULONG NumberOfProcessors; //0xb8
ULONG NtGlobalFlag; //0xbc
union _LARGE_INTEGER CriticalSectionTimeout; //0xc0
ULONGLONG HeapSegmentReserve; //0xc8
ULONGLONG HeapSegmentCommit; //0xd0
ULONGLONG HeapDeCommitTotalFreeThreshold; //0xd8
ULONGLONG HeapDeCommitFreeBlockThreshold; //0xe0
ULONG NumberOfHeaps; //0xe8
ULONG MaximumNumberOfHeaps; //0xec
VOID** ProcessHeaps; //0xf0
VOID* GdiSharedHandleTable; //0xf8
VOID* ProcessStarterHelper; //0x100
ULONG GdiDCAttributeList; //0x108
UCHAR Padding3[4]; //0x10c
struct _RTL_CRITICAL_SECTION* LoaderLock; //0x110
ULONG OSMajorVersion; //0x118
ULONG OSMinorVersion; //0x11c
USHORT OSBuildNumber; //0x120
USHORT OSCSDVersion; //0x122
ULONG OSPlatformId; //0x124
ULONG ImageSubsystem; //0x128
ULONG ImageSubsystemMajorVersion; //0x12c
ULONG ImageSubsystemMinorVersion; //0x130
UCHAR Padding4[4]; //0x134
ULONGLONG ActiveProcessAffinityMask; //0x138
ULONG GdiHandleBuffer[60]; //0x140
VOID (*PostProcessInitRoutine)(); //0x230
VOID* TlsExpansionBitmap; //0x238
ULONG TlsExpansionBitmapBits[32]; //0x240
ULONG SessionId; //0x2c0
UCHAR Padding5[4]; //0x2c4
union _ULARGE_INTEGER AppCompatFlags; //0x2c8
union _ULARGE_INTEGER AppCompatFlagsUser; //0x2d0
VOID* pShimData; //0x2d8
VOID* AppCompatInfo; //0x2e0
struct _UNICODE_STRING CSDVersion; //0x2e8
struct _ACTIVATION_CONTEXT_DATA* ActivationContextData; //0x2f8
struct _ASSEMBLY_STORAGE_MAP* ProcessAssemblyStorageMap; //0x300
struct _ACTIVATION_CONTEXT_DATA* SystemDefaultActivationContextData; //0x308
struct _ASSEMBLY_STORAGE_MAP* SystemAssemblyStorageMap; //0x310
ULONGLONG MinimumStackCommit; //0x318
VOID* SparePointers[4]; //0x320
ULONG SpareUlongs[5]; //0x340
VOID* WerRegistrationData; //0x358
VOID* WerShipAssertPtr; //0x360
VOID* pUnused; //0x368
VOID* pImageHeaderHash; //0x370
union
{
ULONG TracingFlags; //0x378
struct
{
ULONG HeapTracingEnabled:1; //0x378
ULONG CritSecTracingEnabled:1; //0x378
ULONG LibLoaderTracingEnabled:1; //0x378
ULONG SpareTracingBits:29; //0x378
};
};
UCHAR Padding6[4]; //0x37c
ULONGLONG CsrServerReadOnlySharedMemoryBase; //0x380
ULONGLONG TppWorkerpListLock; //0x388
struct _LIST_ENTRY TppWorkerpList; //0x390
VOID* WaitOnAddressHashTable[128]; //0x3a0
VOID* TelemetryCoverageHeader; //0x7a0
ULONG CloudFileFlags; //0x7a8
ULONG CloudFileDiagFlags; //0x7ac
CHAR PlaceholderCompatibilityMode; //0x7b0
CHAR PlaceholderCompatibilityModeReserved[7]; //0x7b1
struct _LEAP_SECOND_DATA* LeapSecondData; //0x7b8
union
{
ULONG LeapSecondFlags; //0x7c0
struct
{
ULONG SixtySecondEnabled:1; //0x7c0
ULONG Reserved:31; //0x7c0
};
};
ULONG NtGlobalFlag2; //0x7c4
};
|
从上面的结构体可以看到0x10偏移处就是镜像的基址: VOID* ImageBaseAddress; 但是如果我想要找到其他模块的基址该如何找呢,放心,PEB可不是这么点东西的。
在ImageBaseAddress紧接着的地方,0x18偏移有意思结构体, _PEB_LDR_DATA* Ldr; 这个结构体就是用来描述当前进程所有装载模块的信息的,它的定义如下:
1
2
3
4
5
6
7
8
9
10
11
12
13
|
//0x58 bytes (sizeof)
struct _PEB_LDR_DATA
{
ULONG Length; //0x0
UCHAR Initialized; //0x4
VOID* SsHandle; //0x8
struct _LIST_ENTRY InLoadOrderModuleList; //0x10
struct _LIST_ENTRY InMemoryOrderModuleList; //0x20
struct _LIST_ENTRY InInitializationOrderModuleList; //0x30
VOID* EntryInProgress; //0x40
UCHAR ShutdownInProgress; //0x48
VOID* ShutdownThreadId; //0x50
};
|
在其中有三个相同类型的结构体,_LIST_ENTRY,这个结构是一个双链表结构的元素,它的定义如下,里面的元素就是前后链接的节点的指针,这样可以“向前”也可以“向后”。
1
2
3
4
5
6
|
//0x10 bytes (sizeof)
struct _LIST_ENTRY
{
struct _LIST_ENTRY* Flink; //0x0
struct _LIST_ENTRY* Blink; //0x8
};
|
再说_PEB_LDR_DATA中的这三个相同结构的元素,每一个元素都代表着一个双链表,而每个链表都绑定的是所有的模块元素,而每一个模块又是相同的元素结构,_LDR_DATA_TABLE_ENTRY,其结构体如下:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
|
//0x120 bytes (sizeof)
struct _LDR_DATA_TABLE_ENTRY
{
struct _LIST_ENTRY InLoadOrderLinks; //0x0
struct _LIST_ENTRY InMemoryOrderLinks; //0x10
struct _LIST_ENTRY InInitializationOrderLinks; //0x20
VOID* DllBase; //0x30
VOID* EntryPoint; //0x38
ULONG SizeOfImage; //0x40
struct _UNICODE_STRING FullDllName; //0x48
struct _UNICODE_STRING BaseDllName; //0x58
union
{
UCHAR FlagGroup[4]; //0x68
ULONG Flags; //0x68
struct
{
ULONG PackagedBinary:1; //0x68
ULONG MarkedForRemoval:1; //0x68
ULONG ImageDll:1; //0x68
ULONG LoadNotificationsSent:1; //0x68
ULONG TelemetryEntryProcessed:1; //0x68
ULONG ProcessStaticImport:1; //0x68
ULONG InLegacyLists:1; //0x68
ULONG InIndexes:1; //0x68
ULONG ShimDll:1; //0x68
ULONG InExceptionTable:1; //0x68
ULONG ReservedFlags1:2; //0x68
ULONG LoadInProgress:1; //0x68
ULONG LoadConfigProcessed:1; //0x68
ULONG EntryProcessed:1; //0x68
ULONG ProtectDelayLoad:1; //0x68
ULONG ReservedFlags3:2; //0x68
ULONG DontCallForThreads:1; //0x68
ULONG ProcessAttachCalled:1; //0x68
ULONG ProcessAttachFailed:1; //0x68
ULONG CorDeferredValidate:1; //0x68
ULONG CorImage:1; //0x68
ULONG DontRelocate:1; //0x68
ULONG CorILOnly:1; //0x68
ULONG ChpeImage:1; //0x68
ULONG ReservedFlags5:2; //0x68
ULONG Redirected:1; //0x68
ULONG ReservedFlags6:2; //0x68
ULONG CompatDatabaseProcessed:1; //0x68
};
};
USHORT ObsoleteLoadCount; //0x6c
USHORT TlsIndex; //0x6e
struct _LIST_ENTRY HashLinks; //0x70
ULONG TimeDateStamp; //0x80
struct _ACTIVATION_CONTEXT* EntryPointActivationContext; //0x88
VOID* Lock; //0x90
struct _LDR_DDAG_NODE* DdagNode; //0x98
struct _LIST_ENTRY NodeModuleLink; //0xa0
struct _LDRP_LOAD_CONTEXT* LoadContext; //0xb0
VOID* ParentDllBase; //0xb8
VOID* SwitchBackContext; //0xc0
struct _RTL_BALANCED_NODE BaseAddressIndexNode; //0xc8
struct _RTL_BALANCED_NODE MappingInfoIndexNode; //0xe0
ULONGLONG OriginalBase; //0xf8
union _LARGE_INTEGER LoadTime; //0x100
ULONG BaseNameHashValue; //0x108
enum _LDR_DLL_LOAD_REASON LoadReason; //0x10c
ULONG ImplicitPathOptions; //0x110
ULONG ReferenceCount; //0x114
ULONG DependentLoadFlags; //0x118
UCHAR SigningLevel; //0x11c
};
|
通过这个结构的定义,尤其是前几个参数就能找到我们需要的内容:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
//0x120 bytes (sizeof)
struct _LDR_DATA_TABLE_ENTRY
{
// 三个结构体,可以找到上一个或者下一个结构体
struct _LIST_ENTRY InLoadOrderLinks; //0x0
struct _LIST_ENTRY InMemoryOrderLinks; //0x10
struct _LIST_ENTRY InInitializationOrderLinks; //0x20
// 模块基址
VOID* DllBase; //0x30
// 模块的入口点函数
VOID* EntryPoint; //0x38
// 镜像的大小
ULONG SizeOfImage; //0x40
// 文件的名称,一个是含绝对路径,一个是纯名称
struct _UNICODE_STRING FullDllName; //0x48
struct _UNICODE_STRING BaseDllName; //0x58
|
既然一样,那为什么会有三个呢,其实通过名字就可以知道,只是顺序不一样,InLoadOrderModuleList 按照加载模块的顺序,InMemoryOrderModuleList内存中模块的顺序,InInitializationOrderModuleList初始化模块的顺序,我们以InLoadOrderModuleList为例做出如下的图,可以简单的更清楚的了解这个关系。
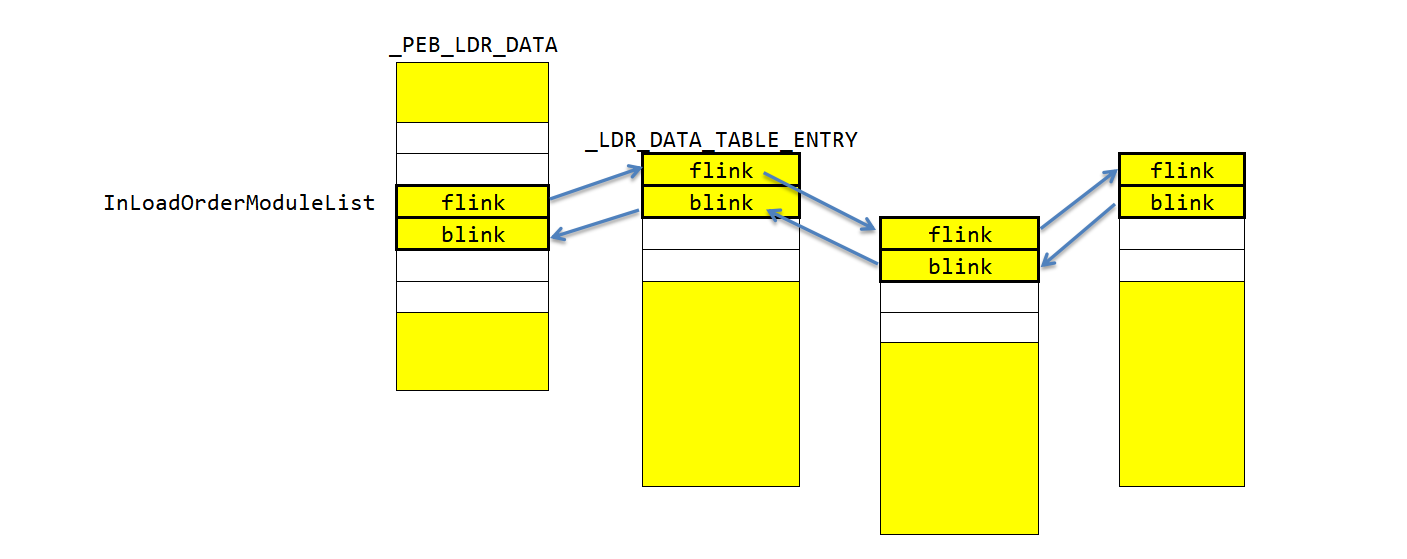
既然知道通过PEB就能找到当前进程以及当前进程导入的所有模块的基址,那剩下的就是找到当前进程的PEB了,windows是提供了找到PEB的一些方法的。
一种方法是我们可以通过TEB来获取PEB,而TEB又是啥,TEB就是线程环境块,就是描述每一个在执行任务的线程的上下文环境的状态,TEB的地址一般就存放在gs/fs的寄存器里,TEB的结构如下问,因为太长不多列出来。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
|
struct _TEB32
{
struct _NT_TIB32 NtTib; //0x0
ULONG EnvironmentPointer; //0x1c
struct _CLIENT_ID32 ClientId; //0x20
ULONG ActiveRpcHandle; //0x28
ULONG ThreadLocalStoragePointer; //0x2c
ULONG ProcessEnvironmentBlock; //0x30
...
copy
//0x1838 bytes (sizeof)
struct _TEB64
{
struct _NT_TIB64 NtTib; //0x0
ULONGLONG EnvironmentPointer; //0x38
struct _CLIENT_ID64 ClientId; //0x40
ULONGLONG ActiveRpcHandle; //0x50
ULONGLONG ThreadLocalStoragePointer; //0x58
ULONGLONG ProcessEnvironmentBlock; //0x60
|
由上面可以看出来,32位系统中,PEB位于0x30位置,64位系统PEB位于0x60
而在x64架构环境下,GS + 0x30处存储的是Teb结构体的基地址;在x86架构环境下,FS + 18h处存储的是Teb结构体的基地址。所以只需要读取寄存器,或者用NtCurrentTeb()
这个方法。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
// 获取当前线程的 TEB
TEB* teb = NtCurrentTeb();
if (teb == nullptr) {
cerr << "Failed to get TEB" << endl;
return 1;
}
// 获取 PEB 的地址
PEB* peb = teb->ProcessEnvironmentBlock;
if (peb == nullptr) {
cerr << "Failed to get PEB" << endl;
return 1;
}
// 打印 PEB 的基址
cout << "PEB base address: " << hex << peb << endl;
|
我们通过x64dbg的操作来可视化获取下对应的值,首先打开一个应用程序,然后在线程标签页中可以找到主线程的TEB,右键复制这个地址,然后到内存窗口。
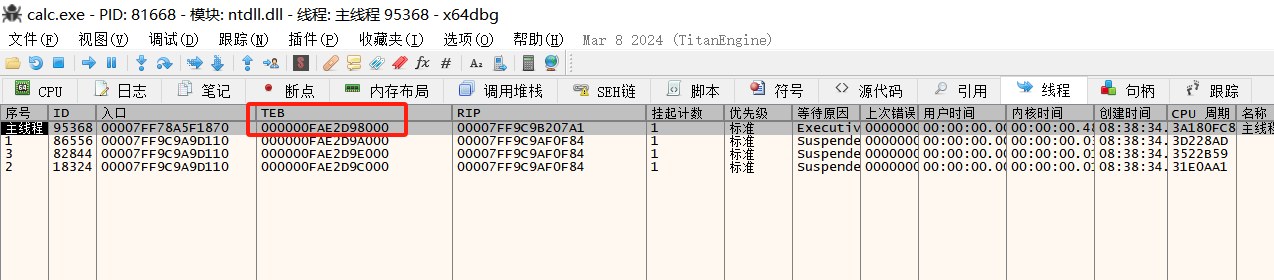
CPU标签页,点击内存窗口地址,ctrl+G,输入复制的teb地址,展示:
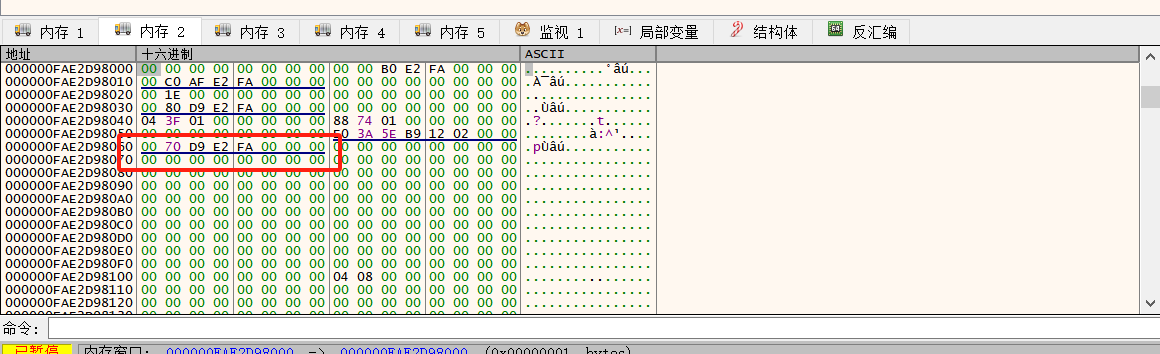
其中0x60位置就是peb地址,我们可以选中8个字节,右键选中在当前内存窗口中转到指定QWORD,也可以选择下面这个,这样可以选择展示在不同的内存窗口,展示:
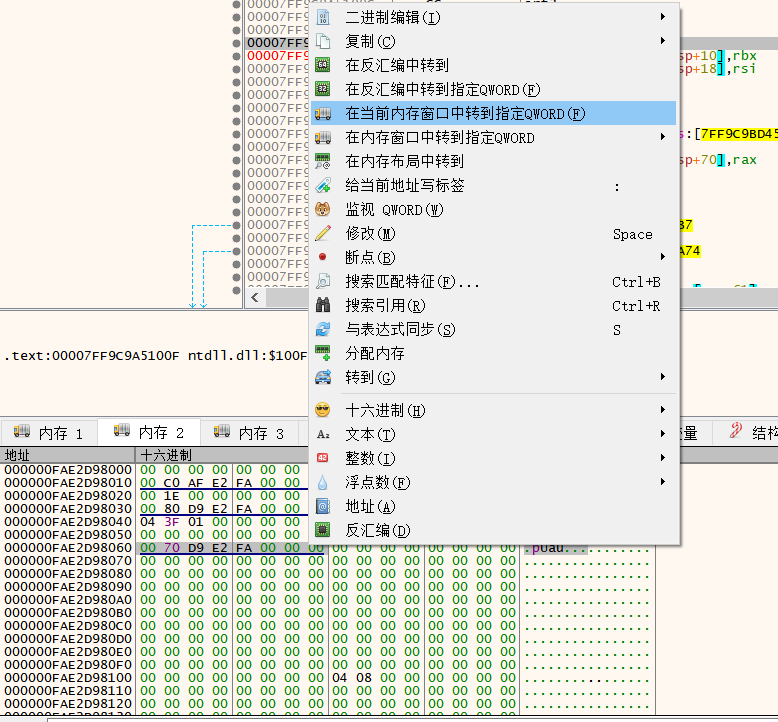
可以看到这个是PEB结构,其中0x10是基址,0x18是_PEB_LDR_DATA的地址,我们依然是选中8个字节,然后右键选择QWORD跳转展示在内存窗口。
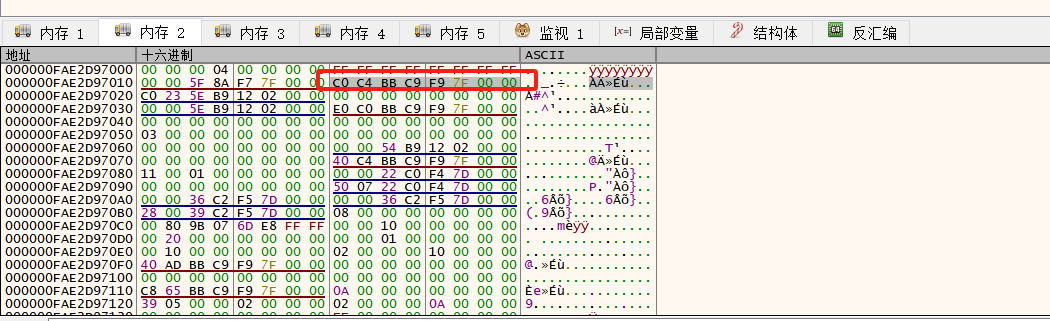
跳转之后如图所以,有三个连续的结构,这应该就是那三个_LIST_ENTRY,我们选择第一个,地址然后依然是选中8个字节,然后右键QWORD,跳转过去。
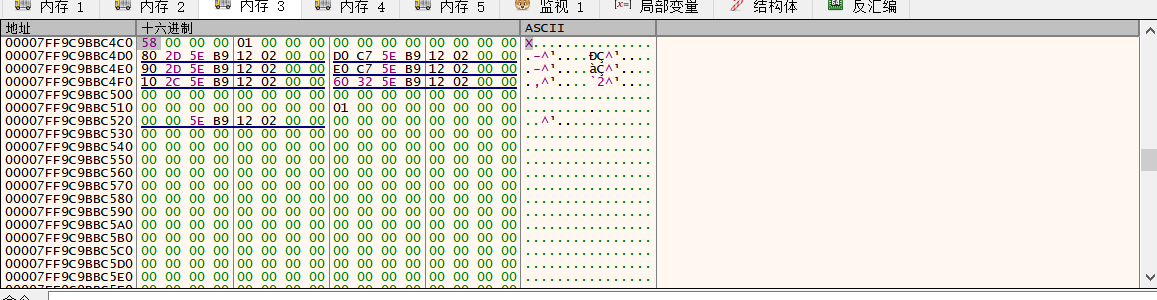
可以看到这样的结构体,应该就是_LDR_DATA_TABLE_ENTRY
,可以看到前面依然是三个一样的结构
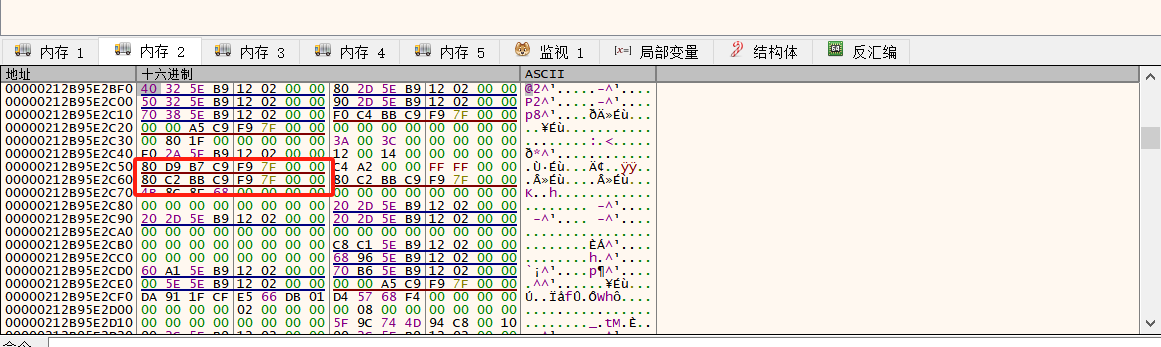
其中,0x48和0x58的位置应该就是就是FullDllName和BaseDllName所对应的UNICODE_STRING
,那么划线的位置应该就是其对应的buffer元素,也就是字符串实际的地址,我们尝试着再次在内存中转过去。
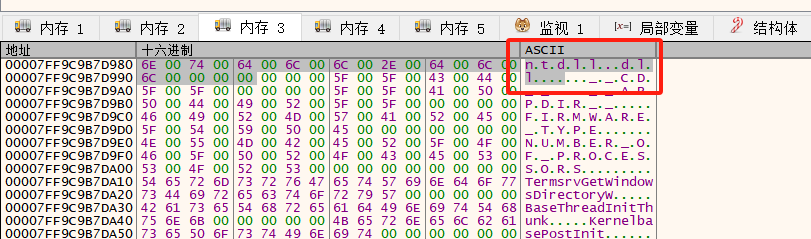
如图所示,可以看到模块的名字,ntdll.dll,也就验证了以上的知识与方法。
但是有个问题,就是其实NtCurrentTeb()
也是利用读取寄存器的方式,可以通过下面的定义看到,但是go对于较底层操作,诸如寄存器的读取是比较麻烦的。
1
2
3
4
5
6
7
8
9
10
11
12
13
|
//////****************
NtCurrentTeb()也是利用读取gs寄存器的值
__forceinline
struct _TEB *
NtCurrentTeb (
VOID
)
{
return (struct _TEB *)__readgsqword(FIELD_OFFSET(NT_TIB, Self));
}
|
所以除了这个函数我们还可以用windows提供的另一个API,NtQueryInformationProcess()
,文档可以看这个链接:
https://learn.microsoft.com/zh-cn/windows/win32/api/winternl/nf-winternl-ntqueryinformationprocess函数的定义如下:
1
2
3
4
5
6
7
|
__kernel_entry NTSTATUS NtQueryInformationProcess(
[in] HANDLE ProcessHandle,
[in] PROCESSINFOCLASS ProcessInformationClass,
[out] PVOID ProcessInformation,
[in] ULONG ProcessInformationLength,
[out, optional] PULONG ReturnLength
);
|
这个函数的调用跟之前我们在驱动里讲的ZwQueryInformationFile
套路相似,https://daliu.net/posts/20241225/#zwqueryinformationfile,所以具体可以参考这个函数的使用。而且这个函数是可以直接获取对应PEB的而不需要TEB了。
但是因为是C语言的的API,所以我们需要用go转化一下,其实是调用,统一就用syscall这个库,这个标准库有很多的方法,都是可以直接调用系统函数,加载dll等
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
// 获取当前进程的句柄
hProcess, _ := syscall.GetCurrentProcess()
// 加载ntdll.dll,
// NewLazyDLL这个方法是懒加载,就是只有当调用方法的时候才会去加载
var ntdll = syscall.NewLazyDLL("ntdll.dll")
// 获取函数指针,可以直接用它调用函数
var NtQueryInformationProcessProc = ntdll.NewProc("NtQueryInformationProcess")
var ProcessBasicInformation PROCESS_BASIC_INFORMATION
var ReturnLength uint32
// 调用函数
ret, _, _ := NtQueryInformationProcessProc.Call(
uintptr(hProcess),
0,
uintptr(unsafe.Pointer(&ProcessBasicInformation)),
uintptr(unsafe.Sizeof(ProcessBasicInformation)),
uintptr(unsafe.Pointer(&ReturnLength)))
|
上述函数执行之后,我们会回去返回值对应的结构体,所以需要解析结构体,获取PEB,同时解析PEB结构体,进一步获取上午提到的一些列参数。但是go也是一个类型严格要求的函数,如果是没有定义的就无法使用,所以我们需要把在对应需要调用参数的结构体提前定义出来:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
|
// 定义UNICODE_STRING,最后打印模块名字的时候使用
type UNICODE_STRING struct {
Length uint16
MaximumLength uint16
Buffer *uint16
}
// _LDR_DATA_TABLE_ENTRY结构体定义,根据Windows的定义
// 后面的没有意义直接省略
type _LDR_DATA_TABLE_ENTRY struct {
InLoadOrderLinks LIST_ENTRY // LIST_ENTRY结构体指针
InMemoryOrderLinks LIST_ENTRY // LIST_ENTRY结构体指针
InInitializationOrderLinks LIST_ENTRY // LIST_ENTRY结构体指针
DllBase uintptr
EntryPoint uintptr
SizeOfImage uint32
FullDllName UNICODE_STRING
BaseDllName UNICODE_STRING
}
// _PEB_LDR_DATA结构体定义,根据Windows的定义
type _PEB_LDR_DATA struct {
Length uint32
Initialized uint8
SsHandle uintptr
InLoadOrderModuleList LIST_ENTRY // LIST_ENTRY结构体指针
InMemoryOrderModuleList LIST_ENTRY // LIST_ENTRY结构体指针
InInitializationOrderModuleList LIST_ENTRY // LIST_ENTRY结构体指针
EntryInProgress uintptr
ShutdownInProgress uint8
ShutdownThreadId uintptr
}
// PEB结构体定义,根据Windows的定义
type PEB struct {
BeingDebugged uint32
Mutant uintptr
ImageBaseAddress uintptr
Ldr *_PEB_LDR_DATA // PEB_LDR_DATA结构体的指针
}
// 这个结构体就是NtQueryInformationProcessProc返回的结构体内容
// 把_PROCESS_BASIC_INFORMATION 转换为go的struct
type PROCESS_BASIC_INFORMATION struct {
ExitStatus uint32
PebBaseAddress *uint64 //peb的指针
AffinityMask uint64
BasePriority int32
UniqueProcessId uint64
InheritedFromUniqueProcessId uint64
}
|
然后就是获取对应的结构体和参数,代码如下:
1
2
3
4
5
6
7
8
9
10
11
12
|
// 读取PEB结构体
peb := (*PEB)(unsafe.Pointer(ProcessBasicInformation.PebBaseAddress))
fmt.Printf("ImageBaseAddress:0x%x\n", peb.ImageBaseAddress)
fmt.Printf("Ldr:0x%x\n", (unsafe.Pointer(peb.Ldr)))
// 读取_PEB_LDR_DATA结构体
ldrPtr := peb.Ldr
fmt.Printf("Length:%d\n", ldrPtr.Length)
fmt.Printf("Initialized:%d\n", ldrPtr.Initialized)
// 读取InLoadOrderModuleList结构体
listEntry := (*_LDR_DATA_TABLE_ENTRY)(unsafe.Pointer(ldrPtr.InLoadOrderModuleList.Flink))
|
当获得基址这个结构体之后其实就已经获得了模块的基址以及模块的名称啥的,那么我们可以写一个循环遍历一个双链表中的模块,然后获取其基址,并用对应方法去解析pe文件,其中读取pe文件的方法我们采用NewFileFromMemory这个方法:
1
2
3
4
5
6
|
// 将uintptr转换为[]byte
dllBytes := unsafe.Slice((*uint8)(unsafe.Pointer(listEntry.DllBase)), listEntry.SizeOfImage)
// 使用通过读取内存的方法获取PE文件信息
ntdll, err := pasepe.NewFileFromMemory(bytes.NewReader(dllBytes))
// 遍历exports
|
获取pe文件之后,剩下的参数读取跟上面的就毫无二致了,把整个读取的代码贴出来大家看下:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
|
package main
import (
"bytes"
"fmt"
"log"
pasepe "github.com/Binject/debug/pe"
"syscall"
"unsafe"
)
func main() {
hProcess, _ := syscall.GetCurrentProcess()
var ntdll = syscall.NewLazyDLL("ntdll.dll")
var NtQueryInformationProcessProc = ntdll.NewProc("NtQueryInformationProcess")
type UNICODE_STRING struct {
Length uint16
MaximumLength uint16
Buffer *uint16
}
// LIST_ENTRY结构体定义,根据Windows的定义
type LIST_ENTRY struct {
Flink *LIST_ENTRY
Blink *LIST_ENTRY
}
// _LDR_DATA_TABLE_ENTRY结构体定义,根据Windows的定义
type _LDR_DATA_TABLE_ENTRY struct {
InLoadOrderLinks LIST_ENTRY // LIST_ENTRY结构体指针
InMemoryOrderLinks LIST_ENTRY // LIST_ENTRY结构体指针
InInitializationOrderLinks LIST_ENTRY // LIST_ENTRY结构体指针
DllBase uintptr
EntryPoint uintptr
SizeOfImage uint32
FullDllName UNICODE_STRING // UNICODE_STRING结构体,此处简化处理为固定长度数组
BaseDllName UNICODE_STRING // 同上,简化处理
}
// _PEB_LDR_DATA结构体定义,根据Windows的定义
type _PEB_LDR_DATA struct {
Length uint32
Initialized uint8
SsHandle uintptr
InLoadOrderModuleList LIST_ENTRY // LIST_ENTRY结构体指针
InMemoryOrderModuleList LIST_ENTRY // LIST_ENTRY结构体指针
InInitializationOrderModuleList LIST_ENTRY // LIST_ENTRY结构体指针
EntryInProgress uintptr
ShutdownInProgress uint8
ShutdownThreadId uintptr
}
// PEB结构体定义,根据Windows的定义
type PEB struct {
BeingDebugged uint32
Mutant uintptr
ImageBaseAddress uintptr
Ldr *_PEB_LDR_DATA // PEB_LDR_DATA结构体的指针
}
// 把_PROCESS_BASIC_INFORMATION 转换为go的struct
type PROCESS_BASIC_INFORMATION struct {
ExitStatus uint32
PebBaseAddress *uint64
AffinityMask uint64
BasePriority int32
UniqueProcessId uint64
InheritedFromUniqueProcessId uint64
}
var ProcessBasicInformation PROCESS_BASIC_INFORMATION
var ReturnLength uint32
ret, _, _ := NtQueryInformationProcessProc.Call(
uintptr(hProcess),
0,
uintptr(unsafe.Pointer(&ProcessBasicInformation)),
uintptr(unsafe.Sizeof(ProcessBasicInformation)),
uintptr(unsafe.Pointer(&ReturnLength)))
fmt.Printf("Return:%d\n", ret)
// 读取PEB结构体
peb := (*PEB)(unsafe.Pointer(ProcessBasicInformation.PebBaseAddress))
fmt.Printf("ImageBaseAddress:0x%x\n", peb.ImageBaseAddress)
fmt.Printf("Ldr:0x%x\n", (unsafe.Pointer(peb.Ldr)))
// 读取_PEB_LDR_DATA结构体,增加类型安全检查
ldrPtr := peb.Ldr
fmt.Printf("Length:%d\n", ldrPtr.Length)
fmt.Printf("Initialized:%d\n", ldrPtr.Initialized)
beginLdr := &(ldrPtr.InLoadOrderModuleList)
// 读取InLoadOrderModuleList结构体
listEntry := (*_LDR_DATA_TABLE_ENTRY)(unsafe.Pointer(ldrPtr.InLoadOrderModuleList.Flink))
for listEntry != nil && &(listEntry.InLoadOrderLinks) != beginLdr {
fmt.Printf("DllBase:0x%x\n", listEntry.DllBase)
fmt.Printf("EntryPoint:0x%x\n", listEntry.EntryPoint)
fmt.Printf("SizeOfImage:%d\n", listEntry.SizeOfImage)
// 使用 unsafe.Slice 创建一个适当大小的 uint16 切片
FullDllName := unsafe.Slice((*uint16)(unsafe.Pointer(listEntry.FullDllName.Buffer)), listEntry.FullDllName.Length/2)
fmt.Printf("FullDllName:%s\n", syscall.UTF16ToString(FullDllName))
baseDllName := unsafe.Slice((*uint16)(unsafe.Pointer(listEntry.BaseDllName.Buffer)), listEntry.BaseDllName.Length/2)
fmt.Printf("BaseDllName:%s\n", syscall.UTF16ToString((baseDllName)))
//解析dll
// 将uintptr转换为[]byte
dllBytes := unsafe.Slice((*uint8)(unsafe.Pointer(listEntry.DllBase)), listEntry.SizeOfImage)
// 使用通过读取内存的方法获取PE文件信息
ntdll, err := pasepe.NewFileFromMemory(bytes.NewReader(dllBytes))
// 遍历exports
if err != nil {
log.Fatalf("Failed to parse PE file: %v", err)
}
// 解析导出表
if exp, err := ntdll.Exports(); err == nil {
fmt.Println("导出函数列表:")
for _, sym := range exp {
fmt.Printf("Ordinal %d, Name: %s, Address: %d, Forward: %s\n", sym.Ordinal, sym.Name, sym.VirtualAddress, sym.Forward)
}
} else {
log.Fatalf("Failed to get exports: %v", err)
}
listEntry = (*_LDR_DATA_TABLE_ENTRY)(unsafe.Pointer(listEntry.InLoadOrderLinks.Flink))
}
}
|
执行之后的结果如图所示。
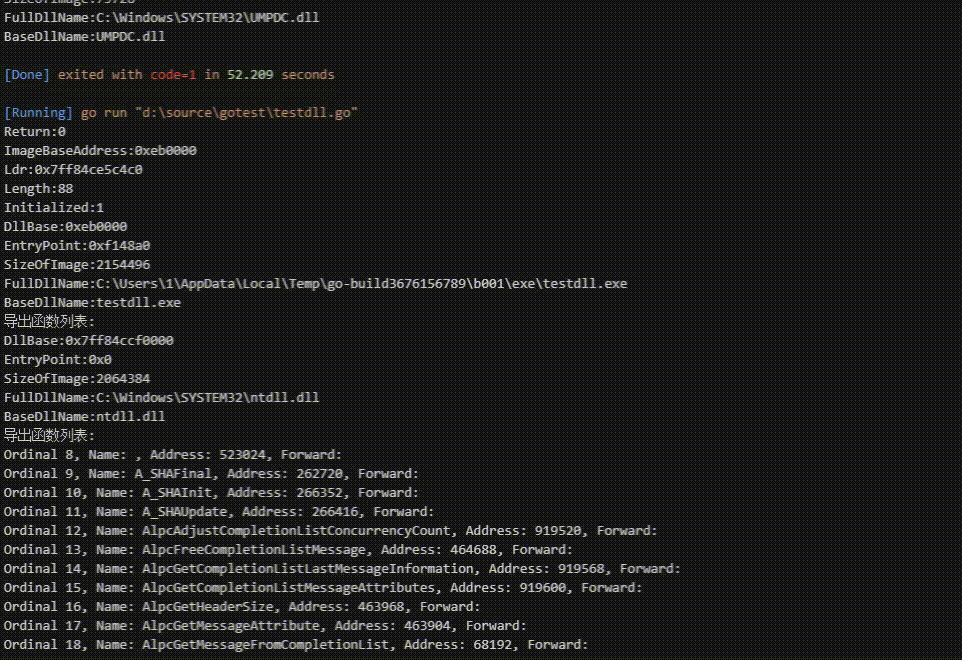
以上就是今天的全部介绍了,我们已经可以通过GO的代码方式实现对pe文件读取并解析,后续可以尝试把几个方法联动,并呈现在页面上。